Mastering Numerical Computing with Julia: A Comprehensive Guide
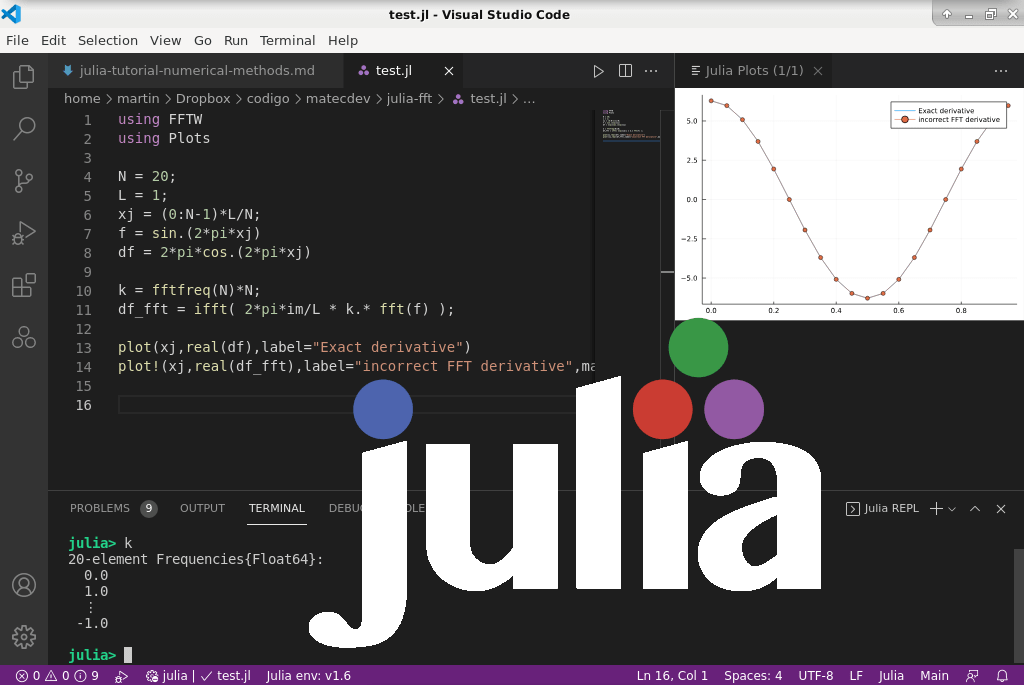
Introduction: Julia is a high-level programming language designed for numerical computing, scientific computing, and data analysis. Known for its speed, flexibility, and ease of use, Julia has gained popularity among researchers, engineers, and data scientists for its ability to combine the productivity of high-level languages like Python with the performance of low-level languages like C or Fortran. In this comprehensive guide, we will explore the intricacies of programming in Julia for numerical computing, covering everything from basic syntax to advanced techniques and optimization strategies.
Section 1: Understanding Julia for Numerical Computing
1.1 Overview of Julia: Julia is a dynamic programming language developed for numerical and scientific computing. It combines features of traditional programming languages with modern conveniences and performance optimizations. Julia is open-source, free to use, and has a growing community of users and developers contributing to its ecosystem.
1.2 Features of Julia: Julia offers several features that make it well-suited for numerical computing:
- High-level syntax: Julia’s syntax is concise, expressive, and easy to read, making it ideal for prototyping and experimentation.
- Multiple dispatch: Julia’s powerful multiple dispatch system allows functions to be defined for combinations of argument types, enabling flexible and efficient code.
- Just-in-time (JIT) compilation: Julia’s JIT compiler translates high-level code to machine code on-the-fly, resulting in performance comparable to statically-typed languages.
- Interoperability: Julia seamlessly integrates with existing libraries and tools written in languages like C, Fortran, and Python, allowing for easy reuse of code and resources.
1.3 Applications of Julia: Julia finds applications in various domains, including:
- Scientific computing: Julia is used for solving mathematical equations, numerical simulations, optimization problems, and computational modeling.
- Data analysis: Julia is employed for processing and analyzing large datasets, performing statistical analysis, and visualizing data using libraries like DataFrames.jl and Plots.jl.
- Machine learning: Julia’s performance and flexibility make it suitable for implementing machine learning algorithms, training models, and building predictive analytics systems.
Section 2: Getting Started with Julia Programming
2.1 Installation: To get started with Julia, download and install the latest version of the Julia programming language from the official website (https://julialang.org/). Follow the installation instructions for your operating system.
2.2 Basic Syntax: Julia’s syntax is similar to other high-level programming languages like Python and MATLAB. Here are some basic syntax elements:
- Variables: Variables are declared using the assignment operator
=
. For example,x = 10
. - Functions: Functions are defined using the
function
keyword. For example,function add(x, y) return x + y end
. - Control flow: Julia supports if-else statements, for loops, while loops, and comprehensions for control flow.
2.3 Data Types: Julia supports various built-in data types, including integers, floating-point numbers, strings, arrays, tuples, dictionaries, and user-defined types. Data types can be declared explicitly or inferred by the compiler based on context.
Section 3: Numerical Computing in Julia
3.1 Arrays and Matrices: Arrays are fundamental data structures in Julia for storing and manipulating numerical data. Julia provides built-in support for arrays, matrices, and vectorized operations. Arrays can be created using the Array
constructor or using array comprehensions.
3.2 Linear Algebra: Julia has a rich set of built-in functions and libraries for linear algebra operations, including matrix multiplication, eigenvalue decomposition, singular value decomposition, and solving systems of linear equations. The LinearAlgebra
standard library provides a comprehensive suite of linear algebra routines.
3.3 Numerical Integration and Differentiation: Julia provides libraries for numerical integration and differentiation, including quadgk for numerical integration and ForwardDiff.jl for automatic differentiation. These libraries enable users to perform numerical analysis tasks such as computing definite integrals and finding derivatives of functions.
Section 4: Advanced Techniques and Optimization Strategies
4.1 Performance Optimization: Julia offers several techniques for optimizing performance, including type annotations, loop fusion, vectorization, and parallel computing. By using these techniques, developers can write code that runs efficiently and takes full advantage of Julia’s performance capabilities.
4.2 Just-In-Time (JIT) Compilation: Julia’s JIT compiler translates high-level Julia code to machine code, optimizing performance on-the-fly. By writing type-stable code and minimizing type instability, developers can improve the efficiency of JIT compilation and achieve faster execution times.
4.3 GPU Computing: Julia supports GPU computing through libraries like CUDA.jl and ROCArray.jl, enabling developers to leverage the computational power of GPUs for numerical computations. By offloading computations to GPUs, developers can achieve significant performance gains for parallelizable tasks.
Section 5: Best Practices and Tips for Julia Programming
5.1 Code Organization: Organize Julia code into modules, functions, and scripts to improve readability, maintainability, and reusability. Use meaningful variable names, comments, and documentation to enhance code clarity and understandability.
5.2 Testing and Debugging: Write unit tests and integration tests to validate the correctness and reliability of Julia code. Use built-in testing frameworks like Test.jl and debugging tools like Juno or VS Code for debugging and troubleshooting code issues.
5.3 Community Engagement: Engage with the Julia community through forums, mailing lists, and social media channels to share knowledge, collaborate on projects, and seek help with programming challenges. Contribute to open-source projects, libraries, and documentation to support the growth and development of the Julia ecosystem.
Conclusion: Programming in Julia for numerical computing offers a powerful and versatile platform for solving complex mathematical problems, performing scientific simulations, and analyzing large datasets. By mastering the principles, techniques, and best practices discussed in this guide, developers can leverage Julia to build efficient, reliable, and scalable numerical computing applications. With its speed, flexibility, and growing ecosystem of libraries and tools, Julia continues to be a preferred choice for researchers, engineers, and data scientists seeking high-performance computing solutions in a dynamic and evolving computational landscape.