Mastering Event Handling in React: A Comprehensive Guide for Effective UI Interaction
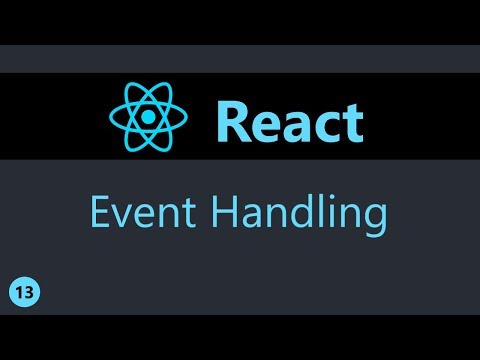
Introduction: Event handling is a fundamental aspect of building interactive user interfaces in React. React provides a declarative and efficient way to manage events, allowing developers to create responsive and dynamic UIs that respond to user actions. In this comprehensive guide, we will explore the principles, techniques, and best practices for handling events in React, empowering developers to create rich and interactive web applications with ease.
- Understanding Events in React: In React, events are actions or occurrences that happen in the user interface, such as mouse clicks, keyboard presses, form submissions, or touch gestures. React abstracts browser events into synthetic events, providing a unified interface for handling events across different browsers and devices. React’s event system follows the same naming conventions as native browser events, making it familiar and easy to use for developers.
- Event Handling Syntax: In React, event handlers are defined as functions that are called when a specific event occurs on a UI element, such as a button click or input change. Event handlers are attached to DOM elements using JSX syntax, where they are passed as props to the corresponding React components. Event handlers can be defined inline as arrow functions or bound to class methods using the
bind()
method in the constructor. - Handling Click Events: Click events are one of the most common types of events in web applications, triggered when the user clicks on a UI element such as a button, link, or image. In React, click events are handled using the
onClick
prop, which takes a function that is called when the element is clicked. Developers can use click events to perform actions such as navigating to a different page, submitting a form, or toggling a UI component’s visibility. - Handling Form Events: Form events are events that occur when the user interacts with HTML form elements such as input fields, checkboxes, radio buttons, and dropdowns. In React, form events such as
onChange
,onSubmit
,onFocus
, andonBlur
are handled using the corresponding event handler props. Form event handlers are used to capture user input, validate input data, and perform form submissions or updates. - Handling Keyboard Events: Keyboard events are events that occur when the user presses or releases keys on the keyboard. In React, keyboard events such as
onKeyDown
,onKeyPress
, andonKeyUp
are handled using the corresponding event handler props. Keyboard event handlers are used to capture user input, implement keyboard shortcuts, navigate through UI elements, and trigger actions based on key presses. - Handling Mouse Events: Mouse events are events that occur when the user interacts with the mouse, such as moving the cursor, clicking, dragging, or scrolling. In React, mouse events such as
onMouseEnter
,onMouseLeave
,onMouseMove
,onMouseDown
,onMouseUp
, andonMouseWheel
are handled using the corresponding event handler props. Mouse event handlers are used to track mouse movements, detect clicks, and implement interactive features such as tooltips, dropdown menus, and sliders. - Handling Touch Events: Touch events are events that occur when the user interacts with the touchscreen on mobile devices, such as tapping, swiping, pinching, or zooming. In React, touch events such as
onTouchStart
,onTouchMove
,onTouchEnd
, andonTouchCancel
are handled using the corresponding event handler props. Touch event handlers are used to create touch-responsive interfaces, support gestures, and optimize the user experience on touch-enabled devices. - Preventing Default Behavior: In some cases, developers may want to prevent the default behavior of an event, such as preventing a form from submitting or preventing a link from navigating to a new page. In React, developers can prevent the default behavior of an event by calling the
preventDefault()
method on the event object passed to the event handler function. Preventing default behavior allows developers to control how events are handled and provide a more intuitive and consistent user experience. - Propagation and Event Bubbling: Event propagation is the process by which events are passed from the target element to its parent elements in the DOM hierarchy. By default, events in React follow the bubbling phase of the event propagation model, where events are first captured by the target element and then propagated up the DOM tree to its ancestors. Developers can control event propagation using the
stopPropagation()
method to stop the event from bubbling up the DOM tree. - Best Practices and Considerations: To effectively handle events in React, developers should follow best practices and considerations such as keeping event handling logic separate from UI rendering logic, using descriptive event handler names, binding event handlers to the correct
this
context, optimizing event performance, and testing event handling behavior thoroughly. Developers should also consider accessibility, device compatibility, and user expectations when designing event-driven UI interactions.
Conclusion: Handling events in React is essential for building interactive and engaging user interfaces that respond to user actions and provide a seamless user experience. By mastering the principles, techniques, and best practices covered in this guide, developers can create rich and dynamic web applications with React that delight users and meet the demands of modern web development. Whether handling click events, form events, keyboard events, mouse events, or touch events, React provides a flexible and efficient event handling system that empowers developers to create intuitive and interactive UIs with ease.