Python Tuples: An In-Depth Exploration
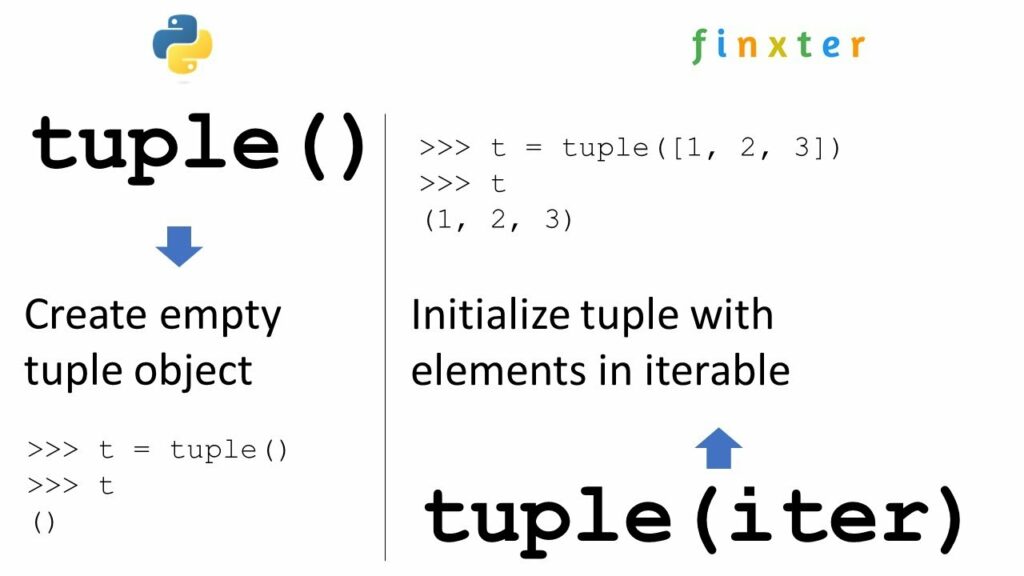
Understanding Tuples
Tuples are immutable sequences in Python. This means that once a tuple is created, its elements cannot be changed. They are often used to store related data items that should not be modified, such as coordinates, dates, or configuration settings.
Creating Tuples
Tuples are created by enclosing a comma-separated sequence of values within parentheses:
my_tuple = (1, 2, 3, "hello", True)
Note that the parentheses are optional in many cases:
my_tuple = 1, 2, 3
Accessing Tuple Elements
You can access individual elements using their index, similar to lists:
first_element = my_tuple[0] # Output: 1
third_element = my_tuple[2] # Output: 3
Python also supports negative indexing for tuples:
last_element = my_tuple[-1] # Output: True
Slicing Tuples
You can extract a portion of a tuple using slicing:
subtuple = my_tuple[1:4] # Output: (2, 3, "hello")
The syntax is similar to list slicing: tuple[start:end:step]
.
Tuple Immutability
As mentioned, tuples are immutable. This means you cannot modify their elements directly:
my_tuple[1] = "world" # This will raise a TypeError
Tuple Length and Membership
Length: Use the
len()
function to determine the number of elements:Pythonlength = len(my_tuple)
Membership: Check if an element exists in the tuple using the
in
keyword:Pythonif "hello" in my_tuple: print("Found!")
Tuple Packing and Unpacking
Packing: Creating a tuple from multiple values:
Pythonx, y, z = 1, 2, 3 # Tuple packing my_tuple = x, y, z
Unpacking: Assigning tuple elements to multiple variables:
Pythona, b, c = my_tuple # Tuple unpacking
Tuple Methods
Tuples have limited methods compared to lists because of their immutability:
count(x)
: Returns the number of occurrences ofx
in the tuple.index(x)
: Returns the index of the first occurrence ofx
.
Using Tuples as Keys in Dictionaries
Tuples can be used as keys in dictionaries because they are immutable and hashable:
my_dict = {(1, 2): "value"}
Tuple Comparisons
Tuples can be compared using comparison operators:
tuple1 = (1, 2, 3)
tuple2 = (2, 1, 3)
tuple3 = (1, 2, 3)
print(tuple1 < tuple2) # Output: True
print(tuple1 == tuple3) # Output: True
Comparison is done element-wise from left to right.
Tuple Advantages
- Efficiency: Tuples are generally faster than lists due to their immutability.
- Security: Protecting data from accidental modification.
- Hashability: Can be used as keys in dictionaries.
When to Use Tuples
- Storing fixed collections of items.
- Returning multiple values from functions.
- Using as keys in dictionaries.
- Representing data that should not be changed.
Common Tuple Operations
- Concatenation: Combining tuples using the
+
operator. - Repetition: Creating a new tuple by repeating an existing tuple using the
*
operator. - Slicing: Extracting a subtuple using the slicing syntax.
Advanced Tuple Topics
- Nested tuples: Tuples can contain other tuples.
- Tuple unpacking with the
*
operator: Unpacking elements into a list. - Tuple comprehensions (less common than list comprehensions).
By understanding these concepts, you can effectively use tuples in your Python programs to improve code readability, performance, and data integrity.