Python Lists: A Comprehensive Guide
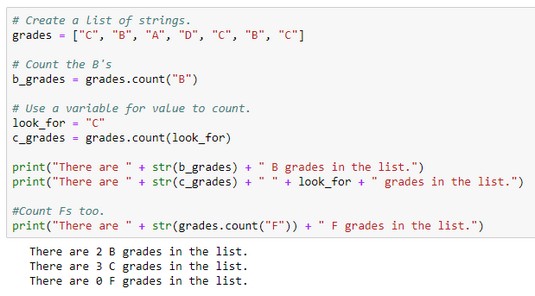
Understanding Python Lists
Python lists are versatile data structures that allow you to store an ordered collection of items. They are mutable, meaning you can change their contents after creation. Lists can hold elements of different data types, making them highly flexible.
Creating Lists
To create a list, enclose a comma-separated sequence of items within square brackets []
:
my_list = [1, 2, 3, "hello", True]
Accessing List Elements
You can access individual elements using their index, starting from 0:
first_element = my_list[0] # Output: 1
third_element = my_list[2] # Output: 3
Python also supports negative indexing, where -1 refers to the last element, -2 to the second-to-last, and so on:
last_element = my_list[-1] # Output: True
Slicing Lists
You can extract a portion of a list using slicing:
sublist = my_list[1:4] # Output: [2, 3, "hello"]
The syntax is list[start:end:step]
, where:
start
is the starting index (inclusive)end
is the ending index (exclusive)step
is the step size (default is 1)
Modifying List Elements
Lists are mutable, so you can change their elements directly:
my_list[1] = "world" # Changes the second element to "world"
Adding Elements to a List
Append: Adds an element to the end of the list:
Pythonmy_list.append(42)
Insert: Inserts an element at a specific index:
Pythonmy_list.insert(2, "new_element")
Extend: Adds multiple elements from another iterable:
Pythonmy_list.extend([5, 6, 7])
Removing Elements from a List
Remove: Removes the first occurrence of a specified value:
Pythonmy_list.remove("hello")
Pop: Removes and returns the element at a specific index:
Pythonremoved_element = my_list.pop(2)
Del: Deletes an element at a specific index:
Pythondel my_list[0]
List Length and Membership
Length: Get the number of elements in a list:
Pythonlength = len(my_list)
Membership: Check if an element is in the list:
Pythonif "hello" in my_list: print("Found!")
List Methods
Python provides numerous built-in methods for list manipulation:
count(x)
: Returns the number of occurrences ofx
in the list.index(x)
: Returns the index of the first occurrence ofx
.sort()
: Sorts the list in ascending order.reverse()
: Reverses the order of elements in the list.copy()
: Returns a shallow copy of the list.
List Comprehensions
List comprehensions offer a concise way to create lists:
squares = [x**2 for x in range(10)]
Nested Lists
Lists can contain other lists, creating nested structures:
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Iterating Over Lists
You can iterate over list elements using a for
loop:
for item in my_list:
print(item)
List Unpacking
You can unpack elements from a list into variables:
a, b, c = [1, 2, 3]
Common List Operations
- Concatenation: Combining two lists using the
+
operator. - Repetition: Creating a new list by repeating an existing list using the
*
operator. - Slicing: Extracting a sublist using the slicing syntax.
- List comprehensions: Creating lists using concise expressions.
Advanced List Topics
- List comprehensions with conditions.
- Generator expressions for memory efficiency.
- List methods for sorting and searching.
- Custom list classes for specialized behavior.
By mastering these concepts, you’ll be well-equipped to effectively use lists in your Python programs.