Python Dictionaries: A Comprehensive Guide
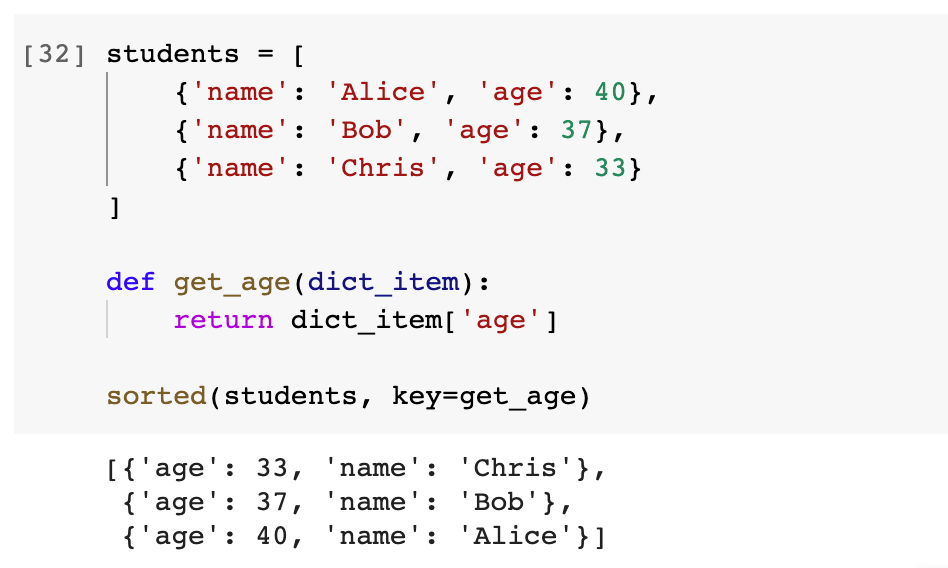
Understanding Python Dictionaries
A Python dictionary is a collection of key-value pairs. It’s an unordered, mutable data structure where each value is associated with a unique key. Dictionaries are incredibly versatile and used extensively in Python programming due to their efficiency in storing and retrieving data.
Creating Dictionaries
To create a dictionary, enclose a comma-separated list of key-value pairs within curly braces {}
:
my_dict = {'name': 'Alice', 'age': 30, 'city': 'New York'}
Accessing Dictionary Values
You access values using their corresponding keys within square brackets:
name = my_dict['name'] # Output: Alice
age = my_dict['age'] # Output: 30
Adding and Modifying Dictionary Elements
Dictionaries are mutable, allowing you to add or modify key-value pairs:
my_dict['occupation'] = 'Engineer' # Add a new key-value pair
my_dict['age'] = 31 # Modify an existing value
Removing Dictionary Elements
You can remove elements using the del
keyword or the pop()
method:
del my_dict['city'] # Remove by key
removed_value = my_dict.pop('occupation') # Remove and return value
Checking for Keys
Use the in
keyword to check if a key exists in the dictionary:
if 'name' in my_dict:
print("Name exists")
Iterating Through a Dictionary
You can iterate over keys, values, or key-value pairs using different methods:
# Iterate over keys
for key in my_dict:
print(key)
# Iterate over values
for value in my_dict.values():
print(value)
# Iterate over key-value pairs
for key, value in my_dict.items():
print(key, value)
Dictionary Methods
Python provides several built-in methods for dictionary manipulation:
clear()
: Removes all items from the dictionary.copy()
: Returns a shallow copy of the dictionary.get(key, default)
: Returns the value for the specified key, or a default value if the key is not found.items()
: Returns a view of the dictionary’s key-value pairs as tuples.keys()
: Returns a view of the dictionary’s keys.popitem()
: Removes and returns an arbitrary key-value pair as a tuple.update(other_dict)
: Updates the dictionary with the key-value pairs from another dictionary or iterable.values()
: Returns a view of the dictionary’s values.
Nested Dictionaries
Dictionaries can contain other dictionaries, creating nested structures:
person = {
'name': 'Alice',
'age': 30,
'address': {
'street': '123 Main St',
'city': 'New York',
'state': 'NY'
}
}
Dictionary Comprehensions
Similar to list comprehensions, dictionary comprehensions provide a concise way to create dictionaries:
squares = {x: x**2 for x in range(5)}
Common Use Cases for Dictionaries
- Storing and retrieving data associated with unique keys.
- Implementing lookup tables.
- Counting occurrences of elements.
- Representing graphs and other data structures.
- Configuring settings and options.
Advanced Dictionary Topics
- Dictionary views: Understand how
keys()
,values()
, anditems()
work. - Defaultdict: Create dictionaries with default values for missing keys.
- OrderedDict: Maintain insertion order of key-value pairs.
- Counter: Count hashable objects efficiently.
- Dictionary performance optimization: Explore techniques for improving dictionary performance.
By mastering dictionaries, you’ll significantly enhance your Python programming skills and be able to tackle a wide range of data-related tasks efficiently.