Mastering Python Threading: A Comprehensive Guide
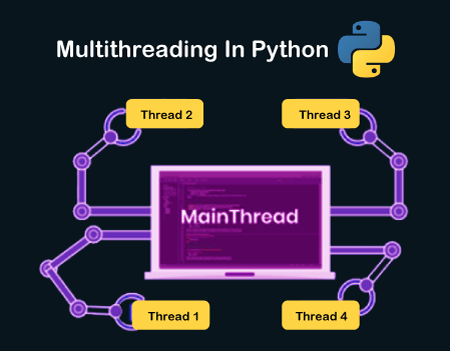
Understanding Threads
Before diving into the threading
module, it’s essential to grasp the concept of threads. A thread is a lightweight process that shares the same memory space as its parent process. Multiple threads can execute concurrently within a single process, allowing for potential performance improvements in I/O-bound tasks.
The threading
Module
Python’s threading
module provides a high-level interface for creating and managing threads.
Creating and Starting Threads
To create a new thread, you typically subclass the threading.Thread
class and override its run()
method.
import threading
import time
def worker():
"""worker function"""
print("Starting thread")
time.sleep(2)
print("Exiting thread")
thread = threading.Thread(target=worker)
thread.start()
The start()
method begins the execution of the thread.
Joining Threads
To wait for a thread to finish, use the join()
method:
thread.join()
print("Main thread finished")
Thread Arguments and Keyword Arguments
You can pass arguments to the target function using the args
and kwargs
parameters of the Thread
constructor:
def worker(name, delay):
# ...
thread = threading.Thread(target=worker, args=("Thread-1", 2))
thread.start()
thread.join()
Daemon Threads
Daemon threads are background threads that automatically terminate when the main program exits.
thread.daemon = True
Thread Local Storage
Thread-local storage (TLS) allows each thread to have its own data that is not shared with other threads.
import threading
local_data = threading.local()
def worker():
local_data.my_data = "Thread-specific data"
print(local_data.my_data)
threads = []
for i in range(5):
t = threading.Thread(target=worker)
threads.append(t)
t.start()
for t in threads:
t.join()
Challenges and Considerations
- Global Interpreter Lock (GIL): Python’s GIL limits the ability of multiple threads to execute Python bytecode at once. This can hinder performance in CPU-bound tasks.
- Race Conditions: Multiple threads accessing shared data can lead to unexpected results. Use locks or other synchronization mechanisms to prevent race conditions.
- Deadlocks: Occur when two or more threads are blocked, waiting for each other to release resources. Avoid circular dependencies and use timeouts to prevent deadlocks.
Synchronization Primitives
Python provides several synchronization primitives to manage thread interactions:
- Locks: Prevent multiple threads from accessing a shared resource simultaneously.
- RLocks: Recursive locks allow a thread to acquire the same lock multiple times.
- Semaphores: Control the number of threads that can access a resource concurrently.
- Events: Provide a way for threads to wait for a specific condition.
- Conditions: More flexible than events, allowing threads to wait for specific conditions and be notified when those conditions change.
Thread Pools
For managing a pool of worker threads, consider using the concurrent.futures
module:
import concurrent.futures
def worker(x):
return x * x
with concurrent.futures.ThreadPoolExecutor(max_workers=4) as executor:
futures = [executor.submit(worker, i) for i in range(10)]
results = [f.result() for f in futures]
When to Use Threading
Threading is most effective for I/O-bound tasks, such as network requests, file operations, or database interactions. It’s generally less beneficial for CPU-bound tasks due to the GIL.
Conclusion
Python’s threading
module provides a foundation for concurrent programming. While it offers advantages in certain scenarios, it’s essential to be aware of its limitations and potential challenges. Careful consideration of thread synchronization and resource management is crucial for building robust and efficient multithreaded applications.