Mastering Python Multiprocessing: A Comprehensive Guide
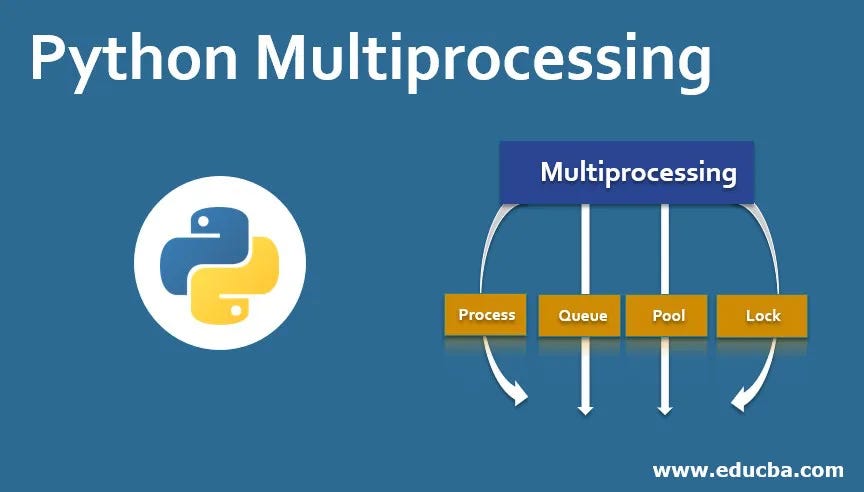
Understanding Multiprocessing
Before diving into the multiprocessing
module, it’s crucial to understand the concept of multiprocessing. Unlike multithreading, which involves multiple threads sharing a single process, multiprocessing involves creating multiple processes, each with its own memory space. This offers distinct advantages, especially for CPU-bound tasks.
The multiprocessing
Module
Python’s multiprocessing
module provides a high-level interface for spawning processes. It offers several ways to achieve parallelism.
Creating and Starting Processes
The most basic way to create a new process is to use the Process
class:
import multiprocessing
def worker():
"""worker function"""
print("Starting process")
# Do some work
print("Exiting process")
if __name__ == "__main__":
p = multiprocessing.Process(target=worker)
p.start()
p.join()
The if __name__ == "__main__":
guard is essential to prevent the creation of multiple processes when the module is imported.
Process Communication
To enable communication between processes, you can use:
- Pipes: Create a pair of connected pipes for inter-process communication.
- Queues: Share data between processes using a queue.
- Managers: Provide a way to manage shared data between processes.
Pipes:
import multiprocessing
def sender(pipe):
pipe.send("Hello, receiver!")
pipe.close()
def receiver(pipe):
msg = pipe.recv()
print(msg)
pipe.close()
if __name__ == "__main__":
parent_conn, child_conn = multiprocessing.Pipe()
p1 = multiprocessing.Process(target=sender, args=(child_conn,))
p2 = multiprocessing.Process(target=receiver, args=(parent_conn,))
p1.start()
p2.start()
p1.join()
p2.join()
Queues:
import multiprocessing
def worker(q):
item = q.get()
print(f"Worker got {item}")
if __name__ == "__main__":
q = multiprocessing.Queue()
p1 = multiprocessing.Process(target=worker, args=(q,))
p1.start()
q.put("Hello")
p1.join()
Managers:
import multiprocessing
def worker(d):
d["x"] = "hi"
if __name__ == "__main__":
manager = multiprocessing.Manager()
d = manager.dict()
p = multiprocessing.Process(target=worker, args=(d,))
p.start()
p.join()
print(d)
Process Pools
For managing a pool of worker processes, use the Pool
class:
import multiprocessing
def worker(x):
return x * x
if __name__ == "__main__":
with multiprocessing.Pool(processes=4) as pool:
results = pool.map(worker, range(10))
print(results)
Sharing Data Between Processes
While sharing data between processes is possible using shared memory or multiprocessing managers, it’s generally more efficient to use process-safe data structures and avoid unnecessary data sharing.
Challenges and Considerations
- Process Creation Overhead: Creating processes is more expensive than creating threads.
- Memory Usage: Each process has its own memory space, which can increase memory consumption.
- Synchronization: Inter-process communication can introduce complexity and potential bottlenecks.
Advanced Topics
- Process Groups: Create groups of processes for coordinated management.
- Process Termination: Control when processes should terminate.
- Error Handling: Implement proper error handling mechanisms for subprocesses.
- Debugging Multiprocessing Code: Use debugging tools effectively to troubleshoot issues.
- Performance Optimization: Optimize multiprocessing code for maximum efficiency.
Conclusion
Python’s multiprocessing
module provides a powerful way to harness multiple cores and improve application performance. By understanding the core concepts, communication mechanisms, and potential challenges, you can effectively utilize multiprocessing to tackle computationally intensive tasks.